July 23, 2017
How to Make an Anti-Spam Voicemail Number With Twilio Functions
SiriusXM keeps calling me because my car apparently came with a free trial, and I gave the dealer my cell number like a dummy. Now the trial is expiring, and they refuse to stop calling me.
There are lots of situations like this: you need to give someone a working phone number, and you most likely won’t want to hear from them. But in rare situations, you will want to – like if my car dealer has to contact me about a safety recall.
What I needed was a special phone number that would discourage telemarketers, but would allow a motivated person to contact me.
Services like Twilio and Plivo make it relatively easy to get a new phone number and allow it to receive calls and record voice messages. But until now there was too much overhead for this to be practical: in addition to the cloud phone service, I would need a separate web server to handle API requests.
This May, Twilio introduced “functions”, a feature that allows users to run custom server-side JavaScript, but without the server. This seemed like a perfect opportunity to create my an anti-spam voicemail number.
Here’s how to set up an anti-spam voicemail number with Twilio functions:
Step 1: Get a phone number
You can do that here for $1/month. You’ll need to set up a Twilio account if you don’t already have one.
Step 2: Set up a function to record a voicemail
Go here, click the +
button, and name your function “Voicemail menu”. Set the path to be /voicemail-menu
.
Here’s the code I used:
exports.handler = function(context, event, callback) {
let actionUrl = 'https://some-subdomain.twil.io/send-sms'
let twiml = new Twilio.twiml.VoiceResponse()
switch (event.Digits) {
case '7':
twiml.say("Please leave a message after the beep. Hang up when finished.");
twiml.record({
'playBeep': true,
'action': actionUrl
});
twiml.hangup();
break
default:
twiml.gather({
numDigits: 1
})
.say('Thank you for calling. If you would like to leave a voicemail, please press 7. Otherwise, you may hang up.')
}
callback(null, twiml)
};
This will read the following in a (pretty good) computer voice when someone calls my number:
Thank you for calling. If you would like to leave a voicemail, please press 7. Otherwise, you may hang up.
If they press 7
, they will hear:
Please leave a message after the beep. Hang up when finished. BEEEEEEP
Otherwise, the call will disconnect.
Step 3: Set up a function to send you an SMS when you get a voicemail
Now you just need a way to get notified of the voicemal and listen to the recording. You can listen via Twilio’s dashboard, but it won’t notify you. My solution: send a text message to my cell phone with a link to the mp3 of the recorded voicemail.
Create a new function here named “Voicemail notifier via SMS” and set the path to be /send-sms
.
Here’s the code I used:
exports.handler = function(context, event, callback) {
twilioClient.messages.create({
to: '+15555555555',
from: event.To,
body: `Voicemail from ${event.From || 'unknown'}: ${event.RecordingUrl || 'no recording'}`
}, callback);
};
Replace +15555555555
with your cell number.
Step 4: Update the URL in Step 2
Grab the path
from your “Voicemail notifier via SMS” function (it should look something like https://something-something-1000.twil.io/send-sms
).
Then open up your “Voicemail menu” function and replace let actionUrl = 'https://some-subdomain.twil.io/send-sms'
with let actionUrl = 'https://YOUR-SUBDOMAIN-GOES-HERE.twil.io/send-sms'
.
Step 5: Attach your function to your phone number
Go here, click your new Twilio phone number, and under “Voice & Fax” choose:
- Accept: incoming voice calls
- Configure with: Webhooks, or TwiML Bins or Functions
- A call comes in: Function / Voicemail menu
Step 6: Test it out
Call your Twilio number from your phone. You should hear the prompt described above. If you press 7 and record a message, you should get a text message like this:
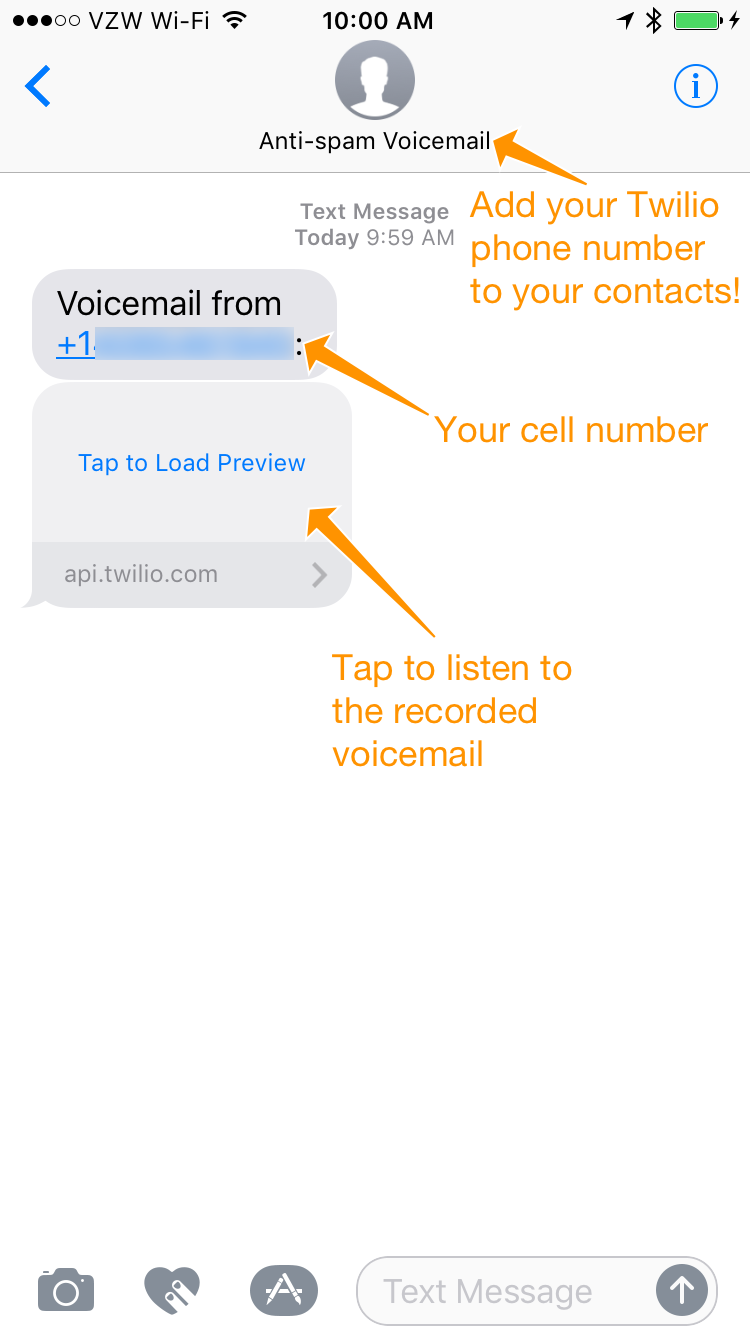
Comments? Please send me a message.